Realm database is growing among developers because of its flexibility and usability, It is more suitable for react native applications rather than using SQLite database because of a proper and stable version. In our previous two tutorials we have discussed about Inserting data into Realm mobile local database and Showing all inserted data into ListView. This tutorial is the next and major part of previous tutorials, In this tutorial we would going to perform basic 4 operations known as Insert, Update, Delete and Display on Realm local mobile database in react native application. So here is the step by step tutorial for Realm Database Insert Update Delete View CRUD Operations in react native android iOS application.
Activities in current project :
- MainActivity : Used to Insert data into Realm mobile database.
- ShowDataActivity : Display all inserted data into ListView.
- EditActivity : Open the selected record filled in TextInput in activity, Also contain the delete button to delete current record.
Contents in this project React Native Realm Database Insert Update Delete View CRUD Operations in iOS Android Example Tutorial:
1. The first step is to install React Navigation library in order to use Activity structure in current application, So open your project’s folder in Command prompt/ Terminal and execute the below command.
1
|
npm install —save react–navigation
|
Screenshot of CMD :

Screenshot of CMD after successfully installed the library:

2. Now we need to install the Realm library in our current to use Realm Local Mobile database in our project, So open react native project folder in CMD and execute the below command.
1
|
npm install —save realm
|
Screenshot of CMD:

Screenshot after successfully installed the Realm library:

3. After installing the Realm library we need to execute
react–native link realm command to re-arrange the complete react native project and index the newly installed realm library.

4. Next step is to start coding, So Open your project’s App.js file and import StyleSheet, Platform, View, Image, Text, TextInput, TouchableOpacity, Alert, YellowBox and ListView component in your project.
1
2
3
|
import React, { Component } from ‘react’;
import { StyleSheet, Platform, View, Image, Text, TextInput, TouchableOpacity, Alert, YellowBox, ListView } from ‘react-native’;
|
5. Import Realm object in your project and make a Global variable named as realm .
1
2
3
|
var Realm = require(‘realm’);
let realm ;
|
6. Import StackNavigator react navigation library in your project to use Activity structure.
1
|
import { StackNavigator } from ‘react-navigation’;
|
7. Create a new class activity named as MainActivity in your project, This would be our first home screen. In this activity we would make 3 TextInput boxes to insert data into Realm mobile database. I have explained all this code in our previous tutorial in Inserting Data Into Realm Mobile database, Read this tutorial.
navigationOptions : Used to set Title of Activity screen.
GoToSecondActivity : Navigate to Second Activity, We would call this function on button onPress event.
constructor() : Inside the constructor we would make 3 States to hold the TextInput entered data, We would also make Table in Realm database.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
|
class MainActivity extends Component{
static navigationOptions =
{
title: ‘MainActivity’,
};
GoToSecondActivity = () =>
{
this.props.navigation.navigate(‘Second’);
}
constructor(){
super();
this.state = {
Student_Name : ”,
Student_Class : ”,
Student_Subject : ”
}
realm = new Realm({
schema: [{name: ‘Student_Info’,
properties:
{
student_id: {type: ‘int’, default: 0},
student_name: ‘string’,
student_class: ‘string’,
student_subject: ‘string’
}}]
});
YellowBox.ignoreWarnings([
‘Warning: componentWillMount is deprecated’,
‘Warning: componentWillReceiveProps is deprecated’,
‘Warning: isMounted(…) is deprecated’, ‘Module RCTImageLoader’
]);
}
add_Student=()=>{
realm.write(() => {
var ID = realm.objects(‘Student_Info’).length + 1;
realm.create(‘Student_Info’, {
student_id: ID,
student_name: this.state.Student_Name,
student_class: this.state.Student_Class,
student_subject : this.state.Student_Subject
});
});
Alert.alert(“Student Details Added Successfully.”)
}
render() {
return (
<View style={styles.MainContainer}>
<TextInput
placeholder=“Enter Student Name”
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Name: text })} }
/>
<TextInput
placeholder=“Enter Student Class”
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Class: text })} }
/>
<TextInput
placeholder=“Enter Student Subject”
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Subject: text })} }
/>
<TouchableOpacity onPress={this.add_Student} activeOpacity={0.7} style={styles.button} >
<Text style={styles.TextStyle}> CLICK HERE TO ADD STUDENT DETAILS </Text>
</TouchableOpacity>
<TouchableOpacity onPress={this.GoToSecondActivity} activeOpacity={0.7} style={styles.button} >
<Text style={styles.TextStyle}> SHOW ALL ENTERED DATA INTO LISTVIEW </Text>
</TouchableOpacity>
</View>
);
}
}
|
Screenshot of MainActivity :
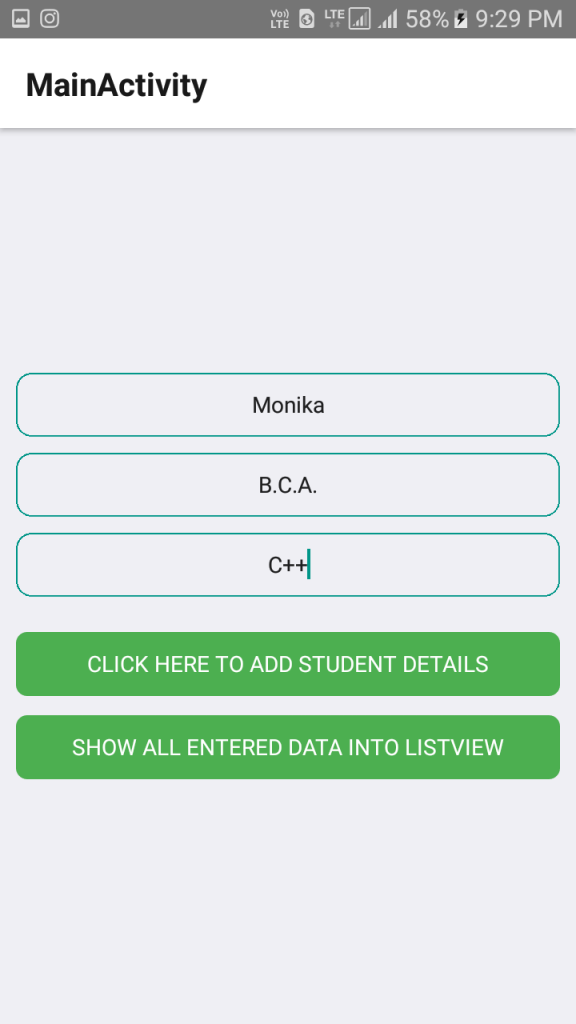
8. Create another class named as ShowDataActivity, This activity parse and show all the inserted Realm Database data into ListView. I have explained all this Activity code into previous tutorial, So read our Show Realm Database Data into ListView tutorial.
1
2
3
4
5
6
|
class ShowDataActivity extends Component
{
}
|
9. Creating navigationOptions in ShowDataActivity to show the activity title.
1
2
3
4
5
6
7
8
|
class ShowDataActivity extends Component
{
static navigationOptions =
{
title: ‘ShowDataActivity’,
};
}
|
10. Creating constructor() inside ShowDataActivity.
YellowBox.ignoreWarnings : To hide the yellow box deprecated warning messages.
mydata : Used to hold all the Student_Info realm database objects.
ds : Setting up ListView data source.
dataSource : Setting up ds in dataSource state.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
constructor() {
super();
YellowBox.ignoreWarnings([
‘Warning: componentWillMount is deprecated’,
‘Warning: componentWillReceiveProps is deprecated’,
‘Warning: isMounted(…) is deprecated’, ‘Module RCTImageLoader’
]);
var mydata = realm.objects(‘Student_Info’);
let ds = new ListView.DataSource({rowHasChanged: (r1, r2) => r1 !== r2});
this.state = {
dataSource: ds.cloneWithRows(mydata),
};
}
|
11. Creating function GoToEditActivity() with 4 parameter student_id, student_name, student_class and student_subject. We would call this function when user clicks on any of Record, this function would open the Third activity and send all 4 selected values to next activity. We are using the this.props.navigation.navigate method to open and send value to next activity.
1
2
3
4
5
6
7
8
9
10
11
12
13
|
GoToEditActivity (student_id, student_name, student_class, student_subject) {
this.props.navigation.navigate(‘Third’, {
ID : student_id,
NAME : student_name,
CLASS : student_class,
SUBJECT : student_subject,
});
}
|
12. Creating function named as ListViewItemSeparator(), This function would make a Horizontal line between each record in ListView.
1
2
3
4
5
6
7
8
9
10
11
|
ListViewItemSeparator = () => {
return (
<View
style={{
height: .5,
width: “100%”,
backgroundColor: “#000”,
}}
/>
);
}
|
13. Creating the ListView component in render’s return block, Inside the ListView we would make 4 TextView to show student details.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
|
render()
{
return(
<View style = { styles.MainContainer }>
<ListView
dataSource={this.state.dataSource}
renderSeparator= {this.ListViewItemSeparator}
renderRow={(rowData) => <View style={{flex:1, flexDirection: ‘column’}} >
<TouchableOpacity onPress={this.GoToEditActivity.bind(this, rowData.student_id, rowData.student_name, rowData.student_class, rowData.student_subject)} >
<Text style={styles.textViewContainer} >{‘id = ‘ + rowData.student_id}</Text>
<Text style={styles.textViewContainer} >{‘Name = ‘ + rowData.student_name}</Text>
<Text style={styles.textViewContainer} >{‘Class = ‘ + rowData.student_class}</Text>
<Text style={styles.textViewContainer} >{‘Subject = ‘ + rowData.student_subject}</Text>
</TouchableOpacity>
</View> }
/>
</View>
);
}
|
14. Complete source code of ShowDataActivity :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
|
class ShowDataActivity extends Component
{
static navigationOptions =
{
title: ‘ShowDataActivity’,
};
constructor() {
super();
YellowBox.ignoreWarnings([
‘Warning: componentWillMount is deprecated’,
‘Warning: componentWillReceiveProps is deprecated’,
‘Warning: isMounted(…) is deprecated’, ‘Module RCTImageLoader’
]);
var mydata = realm.objects(‘Student_Info’);
let ds = new ListView.DataSource({rowHasChanged: (r1, r2) => r1 !== r2});
this.state = {
dataSource: ds.cloneWithRows(mydata),
};
}
GoToEditActivity (student_id, student_name, student_class, student_subject) {
this.props.navigation.navigate(‘Third’, {
ID : student_id,
NAME : student_name,
CLASS : student_class,
SUBJECT : student_subject,
});
}
ListViewItemSeparator = () => {
return (
<View
style={{
height: .5,
width: “100%”,
backgroundColor: “#000”,
}}
/>
);
}
render()
{
return(
<View style = { styles.MainContainer }>
<ListView
dataSource={this.state.dataSource}
renderSeparator= {this.ListViewItemSeparator}
renderRow={(rowData) => <View style={{flex:1, flexDirection: ‘column’}} >
<TouchableOpacity onPress={this.GoToEditActivity.bind(this, rowData.student_id, rowData.student_name, rowData.student_class, rowData.student_subject)} >
<Text style={styles.textViewContainer} >{‘id = ‘ + rowData.student_id}</Text>
<Text style={styles.textViewContainer} >{‘Name = ‘ + rowData.student_name}</Text>
<Text style={styles.textViewContainer} >{‘Class = ‘ + rowData.student_class}</Text>
<Text style={styles.textViewContainer} >{‘Subject = ‘ + rowData.student_subject}</Text>
</TouchableOpacity>
</View> }
/>
</View>
);
}
}
|
Screenshot of ShowDataActivity :
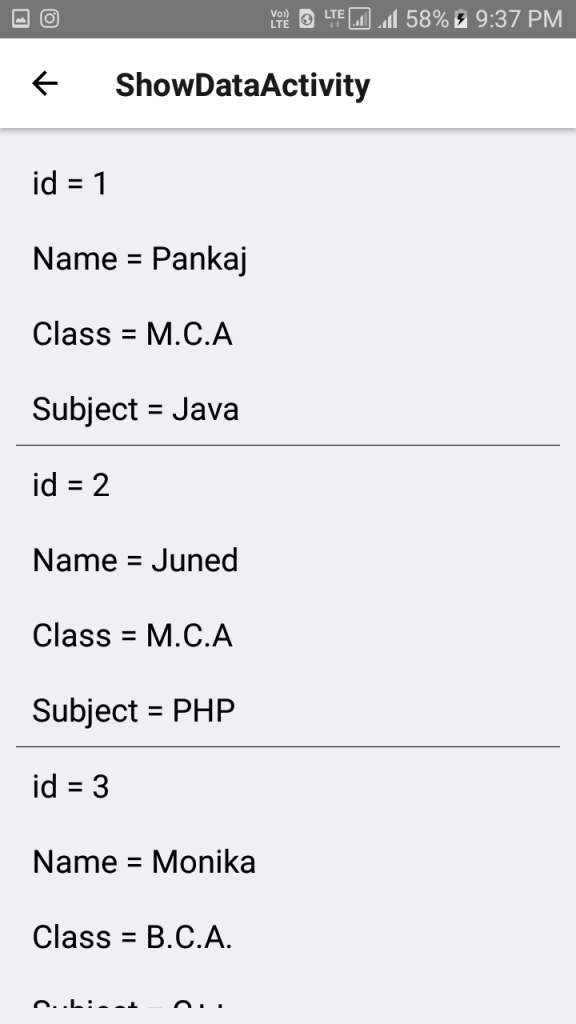
15. Creating the third class named as EditActivity, This class is used to Update the selected record, We would also make a Delete button in class so user would delete the current selected record.
1
2
3
4
5
6
7
8
|
class EditActivity extends Component{
static navigationOptions =
{
title: ‘EditActivity’,
};
}
|
16. Creating constructor() in EditActivity class. Inside the constructor we would make 4 State named as Student_Id, Student_Name, Student_Class and Student_Subject.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
constructor(){
super();
this.state = {
Student_Id : ”,
Student_Name : ”,
Student_Class : ”,
Student_Subject : ”
}
YellowBox.ignoreWarnings([
‘Warning: componentWillMount is deprecated’,
‘Warning: componentWillReceiveProps is deprecated’,
‘Warning: isMounted(…) is deprecated’, ‘Module RCTImageLoader’
]);
}
|
17. Creating the componentDidMount() inbuilt method to receive the send values from previous activity and stored in above created States.
1
2
3
4
5
6
7
8
9
10
11
12
|
componentDidMount(){
// Received Student Details Sent From Previous Activity and Set Into State.
this.setState({
Student_Id : this.props.navigation.state.params.ID,
Student_Name: this.props.navigation.state.params.NAME,
Student_Class: this.props.navigation.state.params.CLASS,
Student_Subject: this.props.navigation.state.params.SUBJECT
})
}
|
18. Creating the Update_Student() function to update the current opened record.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
Update_Student=()=>{
realm.write(() => {
var ID = this.state.Student_Id – 1;
var obj = realm.objects(‘Student_Info’);
obj[ID].student_name = this.state.Student_Name;
obj[ID].student_class = this.state.Student_Class;
obj[ID].student_subject = this.state.Student_Subject;
});
Alert.alert(“Student Details Updated Successfully.”)
}
|
19. Creating the Delete_Student() function to delete the current opened record using current record ID.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
Delete_Student=()=>{
realm.write(() => {
var ID = this.state.Student_Id – 1;
realm.delete(realm.objects(‘Student_Info’)[ID]);
});
Alert.alert(“Record Deleted Successfully.”)
this.props.navigation.navigate(‘First’);
}
|
20. Creating 3 Text Input boxes and 2 buttons in render’s return block.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
|
render() {
return (
<View style={styles.MainContainer}>
<TextInput
value={this.state.Student_Name}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Name: text })} }
/>
<TextInput
value={this.state.Student_Class}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Class: text })} }
/>
<TextInput
value={this.state.Student_Subject}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Subject: text })} }
/>
<TouchableOpacity onPress={this.Update_Student} activeOpacity={0.7} style={styles.button} >
<Text style={styles.TextStyle}> CLICK HERE TO UPDATE STUDENT DETAILS </Text>
</TouchableOpacity>
<TouchableOpacity activeOpacity={0.7} style={styles.button} onPress={this.Delete_Student} >
<Text style={styles.TextStyle}> CLICK HERE TO DELETE CURRENT RECORD </Text>
</TouchableOpacity>
</View>
);
}
|
21. Complete source code of EditActivity class.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
|
class EditActivity extends Component{
static navigationOptions =
{
title: ‘EditActivity’,
};
constructor(){
super();
this.state = {
Student_Id : ”,
Student_Name : ”,
Student_Class : ”,
Student_Subject : ”
}
YellowBox.ignoreWarnings([
‘Warning: componentWillMount is deprecated’,
‘Warning: componentWillReceiveProps is deprecated’,
‘Warning: isMounted(…) is deprecated’, ‘Module RCTImageLoader’
]);
}
componentDidMount(){
// Received Student Details Sent From Previous Activity and Set Into State.
this.setState({
Student_Id : this.props.navigation.state.params.ID,
Student_Name: this.props.navigation.state.params.NAME,
Student_Class: this.props.navigation.state.params.CLASS,
Student_Subject: this.props.navigation.state.params.SUBJECT
})
}
Update_Student=()=>{
realm.write(() => {
var ID = this.state.Student_Id – 1;
var obj = realm.objects(‘Student_Info’);
obj[ID].student_name = this.state.Student_Name;
obj[ID].student_class = this.state.Student_Class;
obj[ID].student_subject = this.state.Student_Subject;
});
Alert.alert(“Student Details Updated Successfully.”)
}
Delete_Student=()=>{
realm.write(() => {
var ID = this.state.Student_Id – 1;
realm.delete(realm.objects(‘Student_Info’)[ID]);
});
Alert.alert(“Record Deleted Successfully.”)
this.props.navigation.navigate(‘First’);
}
render() {
return (
<View style={styles.MainContainer}>
<TextInput
value={this.state.Student_Name}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Name: text })} }
/>
<TextInput
value={this.state.Student_Class}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Class: text })} }
/>
<TextInput
value={this.state.Student_Subject}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Subject: text })} }
/>
<TouchableOpacity onPress={this.Update_Student} activeOpacity={0.7} style={styles.button} >
<Text style={styles.TextStyle}> CLICK HERE TO UPDATE STUDENT DETAILS </Text>
</TouchableOpacity>
<TouchableOpacity activeOpacity={0.7} style={styles.button} onPress={this.Delete_Student} >
<Text style={styles.TextStyle}> CLICK HERE TO DELETE CURRENT RECORD </Text>
</TouchableOpacity>
</View>
);
}
}
|
Screenshot of EditActivity class:
22. Creating StackNavigator activity calling block in order to navigate and manage activities.
1
2
3
4
5
6
7
8
|
export default Project = StackNavigator(
{
First: { screen: MainActivity },
Second: { screen: ShowDataActivity },
Third : { screen: EditActivity}
});
|
23. Creating Style.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
|
const styles = StyleSheet.create({
MainContainer :{
flex:1,
justifyContent: ‘center’,
paddingTop: (Platform.OS) === ‘ios’ ? 20 : 0,
margin: 10
},
TextInputStyle:
{
borderWidth: 1,
borderColor: ‘#009688’,
width: ‘100%’,
height: 40,
borderRadius: 10,
marginBottom: 10,
textAlign: ‘center’,
},
button: {
width: ‘100%’,
height: 40,
padding: 10,
backgroundColor: ‘#4CAF50’,
borderRadius:7,
marginTop: 12
},
TextStyle:{
color:‘#fff’,
textAlign:‘center’,
},
textViewContainer: {
textAlignVertical:‘center’,
padding:10,
fontSize: 20,
color: ‘#000’,
}
});
|
24. Complete source code for App.js File :
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
226
227
228
229
230
231
232
233
234
235
236
237
238
239
240
241
242
243
244
245
246
247
248
249
250
251
252
253
254
255
256
257
258
259
260
261
262
263
264
265
266
267
268
269
270
271
272
273
274
275
276
277
278
279
280
281
282
283
284
285
286
287
288
289
290
291
292
293
294
295
296
297
298
299
300
301
302
303
304
305
306
307
308
309
310
311
312
313
314
315
316
317
318
319
320
321
322
323
324
325
326
327
328
329
330
331
332
333
334
335
336
337
338
339
340
341
342
343
344
345
346
347
348
349
350
351
352
353
354
355
356
357
358
359
360
361
362
363
364
365
366
367
368
369
370
371
372
373
374
375
376
377
378
379
380
381
382
383
384
385
386
387
388
389
390
|
import React, { Component } from ‘react’;
import { StyleSheet, Platform, View, Image, Text, TextInput, TouchableOpacity, Alert, YellowBox, ListView } from ‘react-native’;
var Realm = require(‘realm’);
let realm ;
import { StackNavigator } from ‘react-navigation’;
class MainActivity extends Component{
static navigationOptions =
{
title: ‘MainActivity’,
};
GoToSecondActivity = () =>
{
this.props.navigation.navigate(‘Second’);
}
constructor(){
super();
this.state = {
Student_Name : ”,
Student_Class : ”,
Student_Subject : ”
}
realm = new Realm({
schema: [{name: ‘Student_Info’,
properties:
{
student_id: {type: ‘int’, default: 0},
student_name: ‘string’,
student_class: ‘string’,
student_subject: ‘string’
}}]
});
YellowBox.ignoreWarnings([
‘Warning: componentWillMount is deprecated’,
‘Warning: componentWillReceiveProps is deprecated’,
‘Warning: isMounted(…) is deprecated’, ‘Module RCTImageLoader’
]);
}
add_Student=()=>{
realm.write(() => {
var ID = realm.objects(‘Student_Info’).length + 1;
realm.create(‘Student_Info’, {
student_id: ID,
student_name: this.state.Student_Name,
student_class: this.state.Student_Class,
student_subject : this.state.Student_Subject
});
});
Alert.alert(“Student Details Added Successfully.”)
}
render() {
return (
<View style={styles.MainContainer}>
<TextInput
placeholder=“Enter Student Name”
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Name: text })} }
/>
<TextInput
placeholder=“Enter Student Class”
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Class: text })} }
/>
<TextInput
placeholder=“Enter Student Subject”
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Subject: text })} }
/>
<TouchableOpacity onPress={this.add_Student} activeOpacity={0.7} style={styles.button} >
<Text style={styles.TextStyle}> CLICK HERE TO ADD STUDENT DETAILS </Text>
</TouchableOpacity>
<TouchableOpacity onPress={this.GoToSecondActivity} activeOpacity={0.7} style={styles.button} >
<Text style={styles.TextStyle}> SHOW ALL ENTERED DATA INTO LISTVIEW </Text>
</TouchableOpacity>
</View>
);
}
}
class ShowDataActivity extends Component
{
static navigationOptions =
{
title: ‘ShowDataActivity’,
};
constructor() {
super();
YellowBox.ignoreWarnings([
‘Warning: componentWillMount is deprecated’,
‘Warning: componentWillReceiveProps is deprecated’,
‘Warning: isMounted(…) is deprecated’, ‘Module RCTImageLoader’
]);
var mydata = realm.objects(‘Student_Info’);
let ds = new ListView.DataSource({rowHasChanged: (r1, r2) => r1 !== r2});
this.state = {
dataSource: ds.cloneWithRows(mydata),
};
}
GoToEditActivity (student_id, student_name, student_class, student_subject) {
this.props.navigation.navigate(‘Third’, {
ID : student_id,
NAME : student_name,
CLASS : student_class,
SUBJECT : student_subject,
});
}
ListViewItemSeparator = () => {
return (
<View
style={{
height: .5,
width: “100%”,
backgroundColor: “#000”,
}}
/>
);
}
render()
{
return(
<View style = { styles.MainContainer }>
<ListView
dataSource={this.state.dataSource}
renderSeparator= {this.ListViewItemSeparator}
renderRow={(rowData) => <View style={{flex:1, flexDirection: ‘column’}} >
<TouchableOpacity onPress={this.GoToEditActivity.bind(this, rowData.student_id, rowData.student_name, rowData.student_class, rowData.student_subject)} >
<Text style={styles.textViewContainer} >{‘id = ‘ + rowData.student_id}</Text>
<Text style={styles.textViewContainer} >{‘Name = ‘ + rowData.student_name}</Text>
<Text style={styles.textViewContainer} >{‘Class = ‘ + rowData.student_class}</Text>
<Text style={styles.textViewContainer} >{‘Subject = ‘ + rowData.student_subject}</Text>
</TouchableOpacity>
</View> }
/>
</View>
);
}
}
class EditActivity extends Component{
static navigationOptions =
{
title: ‘EditActivity’,
};
constructor(){
super();
this.state = {
Student_Id : ”,
Student_Name : ”,
Student_Class : ”,
Student_Subject : ”
}
YellowBox.ignoreWarnings([
‘Warning: componentWillMount is deprecated’,
‘Warning: componentWillReceiveProps is deprecated’,
‘Warning: isMounted(…) is deprecated’, ‘Module RCTImageLoader’
]);
}
componentDidMount(){
// Received Student Details Sent From Previous Activity and Set Into State.
this.setState({
Student_Id : this.props.navigation.state.params.ID,
Student_Name: this.props.navigation.state.params.NAME,
Student_Class: this.props.navigation.state.params.CLASS,
Student_Subject: this.props.navigation.state.params.SUBJECT
})
}
Update_Student=()=>{
realm.write(() => {
var ID = this.state.Student_Id – 1;
var obj = realm.objects(‘Student_Info’);
obj[ID].student_name = this.state.Student_Name;
obj[ID].student_class = this.state.Student_Class;
obj[ID].student_subject = this.state.Student_Subject;
});
Alert.alert(“Student Details Updated Successfully.”)
}
Delete_Student=()=>{
realm.write(() => {
var ID = this.state.Student_Id – 1;
realm.delete(realm.objects(‘Student_Info’)[ID]);
});
Alert.alert(“Record Deleted Successfully.”)
this.props.navigation.navigate(‘First’);
}
render() {
return (
<View style={styles.MainContainer}>
<TextInput
value={this.state.Student_Name}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Name: text })} }
/>
<TextInput
value={this.state.Student_Class}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Class: text })} }
/>
<TextInput
value={this.state.Student_Subject}
style = { styles.TextInputStyle }
underlineColorAndroid = “transparent”
onChangeText = { ( text ) => { this.setState({ Student_Subject: text })} }
/>
<TouchableOpacity onPress={this.Update_Student} activeOpacity={0.7} style={styles.button} >
<Text style={styles.TextStyle}> CLICK HERE TO UPDATE STUDENT DETAILS </Text>
</TouchableOpacity>
<TouchableOpacity activeOpacity={0.7} style={styles.button} onPress={this.Delete_Student} >
<Text style={styles.TextStyle}> CLICK HERE TO DELETE CURRENT RECORD </Text>
</TouchableOpacity>
</View>
);
}
}
export default Project = StackNavigator(
{
First: { screen: MainActivity },
Second: { screen: ShowDataActivity },
Third : { screen: EditActivity}
});
const styles = StyleSheet.create({
MainContainer :{
flex:1,
justifyContent: ‘center’,
paddingTop: (Platform.OS) === ‘ios’ ? 20 : 0,
margin: 10
},
TextInputStyle:
{
borderWidth: 1,
borderColor: ‘#009688’,
width: ‘100%’,
height: 40,
borderRadius: 10,
marginBottom: 10,
textAlign: ‘center’,
},
button: {
width: ‘100%’,
height: 40,
padding: 10,
backgroundColor: ‘#4CAF50’,
borderRadius:7,
marginTop: 12
},
TextStyle:{
color:‘#fff’,
textAlign:‘center’,
},
textViewContainer: {
textAlignVertical:‘center’,
padding:10,
fontSize: 20,
color: ‘#000’,
}
});
|
Screenshots: